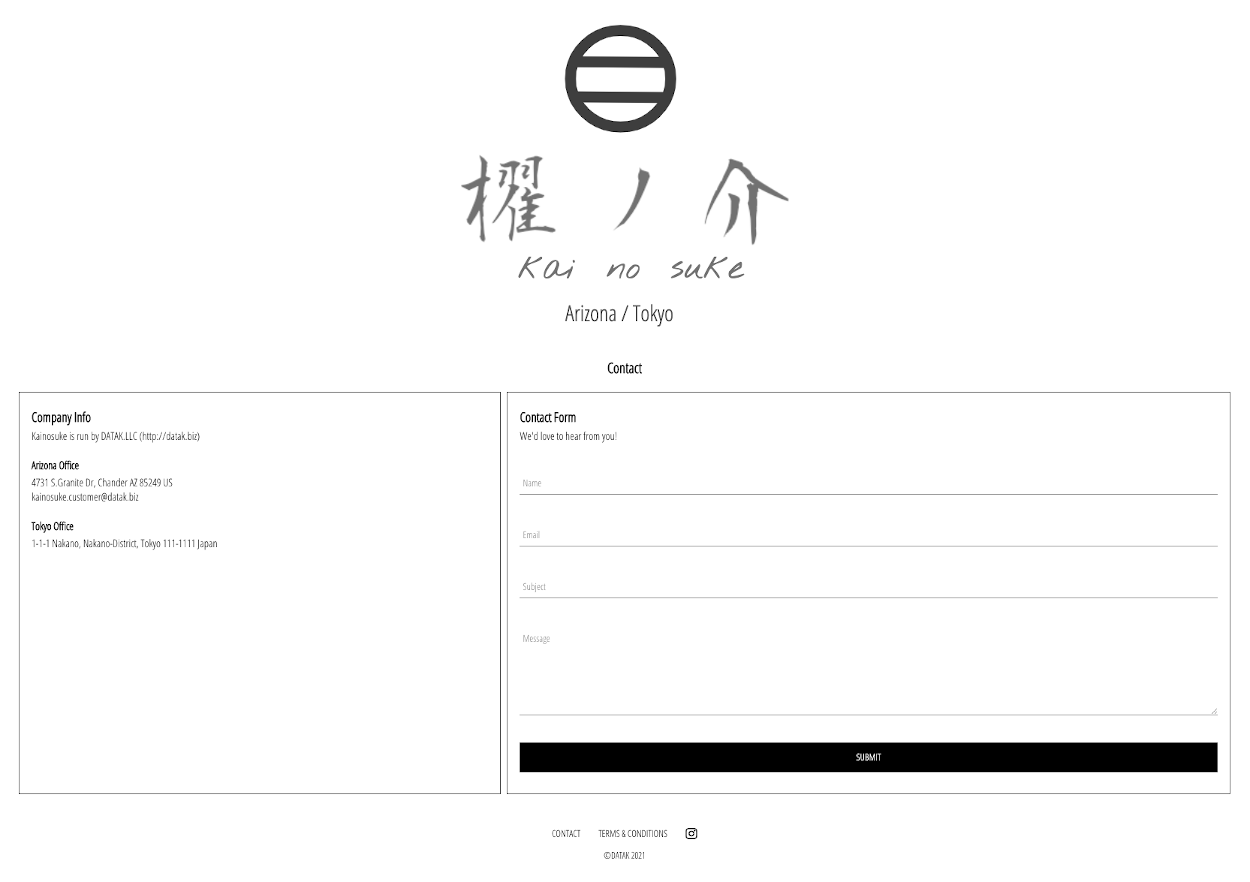
<p>Searching how to integrate contact form into react apps, most of the references I found are to create backend using like node.js with express library, meanwhile I've preferred not to have much backend coding. In the case, using <a href="https://www.emailjs.com/" target="_blank">emailJS</a> would be great candidate, and this post focuses on how to integrate these.</p><p><br></p><h3>Step1 : Create contact form module</h3><p>First let's create new react module only focusing on contact form, <span style="background-color: rgb(245, 245, 245); color: rgb(51, 51, 51); font-family: Menlo, Monaco, Consolas, "Courier New", monospace; font-size: 13px;">contact-us.components.jsx</span> in my case. Also install emailJS dependency at react client folder</p><pre>cd client #in case you've created server.js and split client side react, if not just npm install right under react parent directory npm install emailjs-com --dev</pre><p><br></p><p>Below is overall framing</p><pre>//contact-us.component.jsx import ... //import dependency class ContactUs ... { constructor() { this.state = { //initial state for contact form } } handleSubmit = e => { //event handler when we push submit button and communicate with emailJS library} handleChange = e => { // event handler when user update form } render() { return( //jsx code here for contact form) } }</pre><p><br></p><p>My actual code</p><pre>//contact-us.component.jsx import React from 'react'; import emailjs from 'emailjs-com' import {connect} from 'react-redux'; import {createStructuredSelector} from 'reselect'; import FormInput from '../form-input/form-input.component'; import FormTextArea from '../form-textarea/form-textarea.component'; import { selectCurrentUser } from '../../redux/user/user.selectors'; import {ContactUsContainer, ContactUsButton, SentNotificationText} from './contact-us.styles'; class ContactUs extends React.Component { constructor(){ super(); this.state = { name: '', email: '', subject: '', message: '', submitSuccess: '', } } handleSubmit = e => { e.preventDefault(); // const {name, email, subject, message, submitSuccess} = e.target // e.target automatically includes all variables such as name, email, subject, message, and submitSuccess emailjs.sendForm('service_XXXXXXXXX', 'template_XXXXXXXX', e.target,'user_XXXXXXXXXXXXXXXXXXXX') .then((result) => { console.log(result.text); alert('Your inquiry has been sent. You will get confirmation email shortly') this.resetForm() }, (error) => { console.log(error.text); alert('Something wrong and we could not send your inquery. Please try again later.') }); }; resetForm() { this.setState({ name: '', email: '', subject: '', message: '', submitSuccess: true, }) } handleChange = event => { const {name, value} = event.target; this.setState({ [name]: value }); }; render() { const {name, email, subject, message, submitSuccess} = this.state; return ( <ContactUsContainer> <form onSubmit={this.handleSubmit} id='contact'> <FormInput type='text' name='name' value={name} onChange={this.handleChange} label='Name' required autocomplete='name' /> <FormInput type='email' name='email' value={email} onChange={this.handleChange} label='Email' required autocomplete='email' /> <FormInput type='text' name='subject' value={subject} onChange={this.handleChange} label='Subject' required /> <FormTextArea name='message' value={message} onChange={this.handleChange} label='Message' required form='contact' /> { this.props.currentUser ? <ContactUsButton type='submit'>SUBMIT</ContactUsButton> : <div> <ContactUsButton disabled>SUBMIT</ContactUsButton> <SentNotificationText>In order for us to focus on valuable customers to meet their satisfaction, please log in first</SentNotificationText> </div> } </form> { submitSuccess ? <SentNotificationText>Your inquiry has been submitted. Your will get confirmation email shortly.</SentNotificationText> : null } </ContactUsContainer> ) } } const mapStateToProps = createStructuredSelector({ currentUser: selectCurrentUser }); export default connect(mapStateToProps)(ContactUs);</pre><p><br></p><h3>Step2 : Get emailJS account and API</h3><p>Let's sing up emailJS, you need couple of API key from there;</p><ul><li>Service ID : which email service/provide you use</li><li>Template ID : email template that you create for contact communication with admin</li><li>User ID : your ID</li></ul><p><br></p><h3>Step3: Create your email template in emailJS</h3><p>Go to 'Email Templates' at left pane in emailJS, create your email template to admin. <span style="background-color: rgb(245, 245, 245); color: rgb(51, 51, 51); font-family: Menlo, Monaco, Consolas, "Courier New", monospace; font-size: 13px;">{{your variable}}</span> are variable you could connect to your contact form. Note, some of variable name are taken in emailJS original variable in the template. For example, <span style="background-color: rgb(245, 245, 245); color: rgb(51, 51, 51); font-family: Menlo, Monaco, Consolas, "Courier New", monospace; font-size: 13px;">{{to_name}}</span> is your emailJS account name</p><p><img src="/media/django-summernote/2021-01-10/f7616bec-57c0-4547-8158-573dd38e68fc.png" style="width: 100%;"></p><p><br></p><p><br></p>
<< Back to Blog Posts
Back to Home