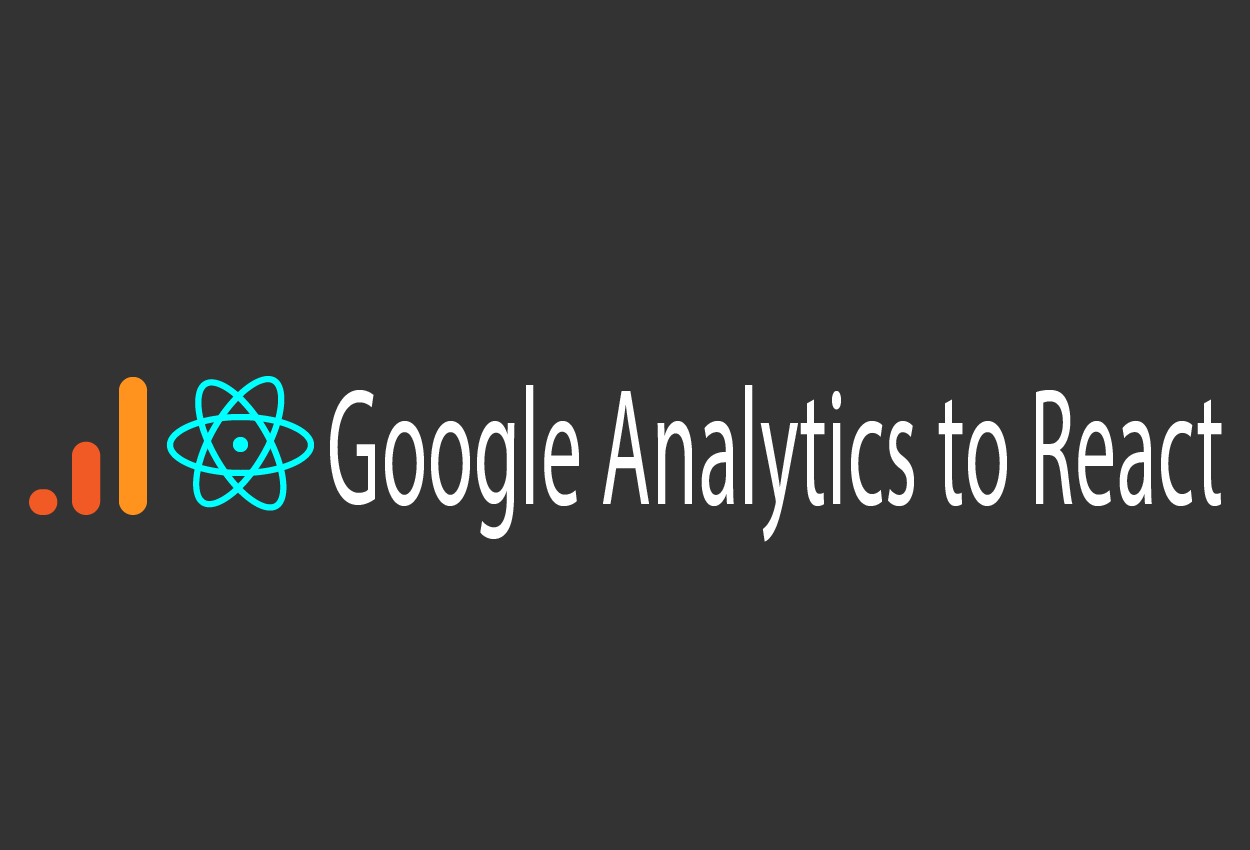
<p>Google analytics lets you easily analyze user action, visualizing key indicators on user action such as traffic, page view, and conversion rate. It typically straightforward to implement, but it is a bit complicated in case we would like to implement to React apps because of its concept - single page application.</p><p>In this article, we will introduce 2 cases to implement page view against differences on Router setting and event tracking.</p><p><br></p><h3>Google Analytics Account Set Up</h3><ul><li>Set up initial account</li><li>Go to Admin at left pane, and create new property for a certain react project that we would like to implement google analytics</li><li>For a property, we will stick with legacy solution (most popular solution as of Jan, it seems that google analytics offering GA4 (app + web) but legacy way still covers most in terms of web application), so push advanced options at step1 of property setup and check "create a universal analytics property" and select radio button for "Create a universal analytics property only"</li></ul><p><img src="/media/django-summernote/2021-01-14/40c54c8e-f4a4-4a2d-968b-eff661ebfa25.png" style="width: 100%; float: none;" class=""></p><ul><li>At step2 prompt, put whatever your business info</li><li>Then you will get "tracking ID" and copy that (we will paste to react code later)</li></ul><p><br></p><h3>Initial Google Analytics Set Up in React App</h3><p>Install google analytics library</p><pre>npm install react-ga --save</pre><p>Go to App.js and put below code</p><pre># react hooks case useEffect(()=>{ ...other code here ReactGA.initialize('your tracking ID here'); },[])</pre><p>or</p><pre style="line-height: 1.42857;"># traditional class module case componentDidMount() { ...other code here ReactGA.initialize('your tracking ID here'); }</pre><p style="line-height: 1.42857;"><br></p><h3>Page View Implementation</h3><p>We have 2 options here and if we have <Router> that is easy, but <Router> is not recommended to use as react router v4 and might have an issue with redux (I got the issues and ended up not using this option)</p><p><br></p><h4>Case1 : Use Router</h4><p>Find your react component where we have Route info like,</p><pre>#your javascript file import {Router, Switch, Route} from 'react-router-dom'; const RouteManagement = () => { ... return( <Router> <Switch> <Route /> <Route /> <Route /> </Switch> </Router> ) }</pre><p>then change to with installing history library;</p><pre>npm install history</pre><pre style="line-height: 1.42857;">#your javascript file import {Router, Switch, Route} from 'react-router-dom'; import {createBrowser} from 'history' const RouteManagement = () => { ... const history = createBrowserHistory(); history.listen(location => { ReactGA.pageview(location.pathname); //console.log(location.pathname); }); return( <Router history={history}> <Switch> <Route /> <Route /> <Route /> </Switch> </Router> ) }</pre><p><br></p><h4>Case2 : Use BrowserRouter</h4><p>Since <Router> is not recommended to latest react router and potentialy compatibility issues with redux, case 2 is to show how to use <BrowserRouter>. In that case, one of the headache is that BrowserRouter does not have history as an argument.</p><p><a href="https://github.com/react-ga/react-ga" target="_blank">At react-ga github page</a>, they put a <a href="https://github.com/react-ga/react-ga/tree/master/demo" target="_blank">demo example</a> and they've created a separate jsx file only for pageview implementation, and it'll be like;</p><pre>//new jsx file called "withTracker.jsx" /** * From ReactGA Community Wiki Page https://github.com/react-ga/react-ga/wiki/React-Router-v4-withTracker */ import React, { Component } from 'react'; import ReactGA from '../../src'; export default function withTracker(WrappedComponent, options = {}) { const trackPage = (page) => { ReactGA.set({ page, ...options }); ReactGA.pageview(page); }; const HOC = class extends Component { componentDidMount() { const { location: { pathname: page } } = this.props; trackPage(page); } // eslint-disable-next-line camelcase UNSAFE_componentWillReceiveProps(nextProps) { const { location: { pathname: currentPage } } = this.props; const nextPage = nextProps.location.pathname; if (currentPage !== nextPage) { trackPage(nextPage); } } render() { return <WrappedComponent {...this.props} />; } }; return HOC; } </pre><div>If you use <ReactStrict> at index.js then react might not recommend to use with componentWillReceiveProps and I've commented out that block. Once we have withTracker.jsx file, go back to your router javascript file, and implement;</div><pre>#your javascript file import {BrowserRouter, Switch, Route} from 'react-router-dom'; import withTracker from '../../google-analytics/withTracker'; const RouteManagement = () => { ... return( <BrowserRouter> <Switch> <Route component={withTracker(homePage)}/> <Route component={withTracker(contactPage} /> <Route component={withTracker(infoPage} /> </Switch> </BrowserRouter> ) }</pre><p><br></p><h3>Event Implementation</h3><p>Find your event handler, and put following code;</p><pre><button onClicke={()=>{ ReactGA.event({ category: 'your category' action: 'your action description' }) }}</pre><p><br></p><p>That's it. Finally you will see the user behavior in google analytics site like this;</p><p>Pageview</p><p><img src="/media/django-summernote/2021-01-14/044de19b-d6ef-47a0-9388-35d76450e3f0.png" style="width: 100%;"></p><p><br></p><p>Event</p><p><img src="/media/django-summernote/2021-01-14/11c96fc4-b0d9-40f6-bffe-00dcf09bd71d.png" style="width: 100%;"><br></p>
<< Back to Blog Posts
Back to Home