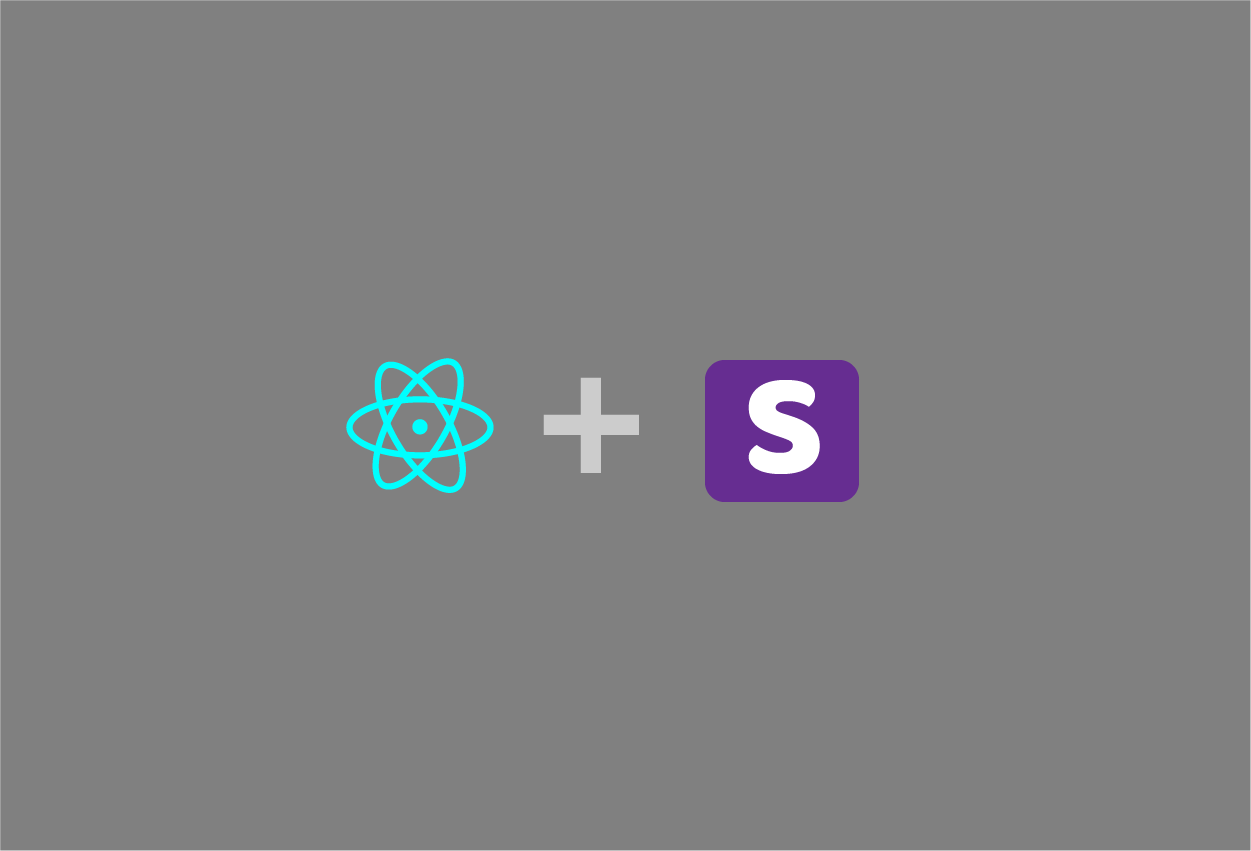
<p>This article includes from explaining stripe through implementing stripe to react application using 'react-stripe-checkout' which is wrapper to use stripe API.</p><p><br></p><h3>What is stripe payments?</h3><blockquote><p style="-webkit-font-smoothing: antialiased; text-rendering: optimizelegibility; letter-spacing: 0.2px; color: rgb(51, 51, 51); font-size: 18px; line-height: 1.9; font-family: "Open Sans", Helvetica, Arial, sans-serif, sans-serif;">Stripe was founded in 2010 with the mission of making it easier to accept payments over the internet. At the time, taking credit cards meant working with a legacy processor or a middleman broker who would provide you with access to a processor. </p><p style="-webkit-font-smoothing: antialiased; text-rendering: optimizelegibility; letter-spacing: 0.2px; color: rgb(51, 51, 51); font-size: 18px; line-height: 1.9; font-family: "Open Sans", Helvetica, Arial, sans-serif, sans-serif;">Then there were the banks you had to deal with, the credit card companies themselves, and a slew of additional services that were often poorly explained and sold by various third parties. Long story short, accepting credit cards was an incredibly complicated and poorly explained process.<br></p><p style="-webkit-font-smoothing: antialiased; text-rendering: optimizelegibility; letter-spacing: 0.2px; color: rgb(51, 51, 51); font-size: 18px; line-height: 1.9; font-family: "Open Sans", Helvetica, Arial, sans-serif, sans-serif;">Stripe set out to fix a lot of that by streamlining the process. They added services and features like fraud protection, fixed rates regardless of network, and an application programming interface (API) that allowed app makers to easily incorporate card processing into their apps.<br><br>reference : <a href="https://www.jotform.com/what-is-stripe/#:~:text=The%20basic%20version%20of%20Stripe,%2C%20JCB%2C%20and%20Diners%20Club." target="_blank">what is stripe payments?</a></p></blockquote><p><br></p><h3>Step1: Set up stripe site</h3><p>Create your account at <a href="https://stripe.com" target="_blank">stripe.com</a>. Your email address in profile is used to get a notification on successful payments. Going to left pane => developers => API keys. Before you activate your account connecting to your business bank account and EIN, you can only use test mode but API keys are only difference in code.</p><p><img src="/media/django-summernote/2021-02-03/769007f6-c927-4cc8-b654-4dcdc203b129.png" style="width: 100%;"><br></p><p><br></p><h3>Step2: Stripe front end</h3><p>Create stripe button component, passing data which you would like to send stripe server.</p><pre>#stripe-button.component.jsx import React from 'react'; import StripeCheckout from 'react-stripe-checkout'; import axios from 'axios'; import { withRouter } from 'react-router-dom'; const StripeCheckoutButton = ({ price, history, cartItems }) => { //stripe needs by cents instead of dollars, while price is based on dollars const priceForStripe = price * 100; const publishableKey = '<your publishable API keys here>'; //token is used to communicate to backend server to charge actual payment const onToken = token => { axios({ url: 'payment', //append 'payment' to create a url to send method: 'post', data: { amount: priceForStripe, token } }).then(response => { alert('Payment Successful. We will proceed your order, and you will receive confirmation email once item is shipped. Thank you for your shopping!'); ////your post successful event here//// }).catch(error => { console.log(error.message); alert('There was an issue with your payment. Please make sure you use the provided credit cart'); }) } return ( <StripeCheckout name='Mionosuke' billingAddress shippingAddress image='https://<your icon image link here>' description={`Your total is $${price}`} amount={priceForStripe} panelLabel='Pay Now' token={onToken} stripeKey={publishableKey} ComponentClass='div' > <Button>PROCEED PAYMENT</Button> </StripeCheckout> ); }; export default StripeCheckoutButton; </pre><p><br></p><p>For a simple integration, we are using react library of 'react-stripe-checkout', which is a wrapper to integrate stripe connection just using <StripeCheckout />.</p><pre>#shellscript npm install --save react-stripe-checkout</pre><p>We need axios library to use url to communicate information to backend server.</p><pre>#shellscript npm install --save axios</pre><p><br></p><h3>Step3: Stripe back end</h3><p>In order to communicate user credit card information, we need a server. So if you create only front end react app, you need to create front end side directory(to be called as 'client') and copy all current react files.</p><pre>#shellscript at parent directory mkdir client cp <all files> client/<all files></pre><p><br></p><p>We are using express server. We need couple of libraries installed.</p><pre>#server.js // in order for charge safe, stripe secret key needs //to be integrated into backend, front end can't encrypt secret key // old version of import const express = require('express'); const cors = require('cors'); const bodyParser = require('body-parser'); const path = require('path'); const enforce = require('express-sslify'); if (process.env.NODE_ENV !== 'production') require('dotenv').config(); const stripe = require('stripe')(process.env.STRIPE_SECRET_KEY); //library to process backend node server const app = express(); const port = process.env.PORT || 5000; app.use(bodyParser.json()); app.use(bodyParser.urlencoded({ extended: true })); //for development server app.use(cors()); //set how to server an application in production if (process.env.NODE_ENV === 'production') { app.use(enforce.HTTPS({ trustProtoHeader: true })); app.use(express.static(path.join(__dirname, 'client/build'))); app.get('*', function(req, res) { res.sendFile(path.join(__dirname, 'client/build', 'index.html')); }); } app.listen(port, error => { if (error) throw error; console.log('Server running on port ' + port); }); //client side send token thru /payment app.post('/payment', (req, res) => { //req object const body = { source: req.body.token.id, //token amount: req.body.amount,//total amount currency: 'usd' }; stripe.charges.create(body, (stripeErr, stripeRes) => { if(stripeErr) { res.status(500).send({ error: stripeErr }); //500 failure status } else { res.status(200).send({ success: stripeRes }); //200 no failure } }) }) </pre><p><br></p><h3>Step4: Deploy</h3><p>We need a server has stripe secret key to communicate with stripe server, but we can't expose the key. Create .env file and put secret key info and clarify .env file in .gitignore. All other deployment process please refer previous post <a href="https://datak.biz/blog/detail/26/" target="_blank">here</a></p>
<< Back to Blog Posts
Back to Home